Step-by-step Guide: How to Create a Volto Addon for Plone
Volto is a modern and powerful frontend for Plone, a popular open-source content management system (CMS). It allows developers to create rich and interactive websites and applications using React, a popular JavaScript library for building user interfaces.
In this tutorial, we will learn how to create a Volto addon for Plone. An addon is a package that extends the functionality of Volto by adding new features or modifying existing ones. Addons can be used to customize the look and feel of a website, add new features to the user interface, or integrate with external services.
Prerequisites:
Before we begin, you should have the following things installed and set up:
- Node.js and npm: These are required to install and run Volto and its dependencies. You can download them from the official website (https://nodejs.org/en/download/).
- Plone: You will need a Plone instance to test your addon. You can either install Plone on your own server or locally.
- Volto: You will need to install Volto to develop and test your addon. You can do this by running the following command:
npm install -g yo @plone/generator-volto
Creating a new addon:
To create a new Volto addon, we will use the Yeoman generator provided by the Plone team. Yeoman is a tool that helps developers generate the skeleton of a new project, saving them time and effort.
To create a new addon, run the following command:
yo @plone/volto:addon
You will be asked to provide a name for your addon. Choose a descriptive and unique name that follows the Plone naming conventions (e.g., “my-addon”).
This will generate a new folder with the same name as your addon, containing the files and directories needed to start developing your addon.
Structure of a Volto addon:
Let’s take a look at the structure of a Volto addon:
my-addon/
+-- CHANGES.rst
+-- CONTRIBUTORS.rst
+-- LICENSE.txt
+-- README.rst
+-- package.json
+-- src/
¦ +-- index.js
¦ +-- index.test.js
¦ +-- my-addon.js
¦ +-- my-addon.test.js
¦ +-- redux
¦ +-- sagas
¦ +-- selectors
¦ +-- styles
¦ +-- views
+-- test-setup.js
Here’s a brief description of each file and directory:
- CHANGES.rst: This file lists the changes made to the addon in each version.
- CONTRIBUTORS.rst: This file lists the contributors to the addon.
- LICENSE.txt: This file contains the license under which the addon is distributed.
- README.rst: This file contains a brief description of the addon and instructions on how to use it.
- package.json: This file contains metadata about the addon, such as its name, version, dependencies, and scripts.
- src/: This directory contains the source code of the addon.
- src/index.js: This file is the entry point of the addon. It exports the main functions and components of the addon.
- src/index.test.js: This file contains the test cases for the functions and components exported by the index.js file.
- src/my-addon.js: This file contains the main functionality of the addon.
- src/my-addon.test.js: This file contains the test cases for the functions and components in my-addon.js.
- src/redux/: This directory contains the Redux-related files of the addon, such as actions, reducers, and store configuration.
- src/sagas/: This directory contains the Redux-Saga files of the addon, which handle side effects and asynchronous logic.
- src/selectors/: This directory contains the selector functions of the addon, which are used to extract data from the Redux store.
- src/styles/: This directory contains the CSS files of the addon.
- src/views/: This directory contains the React components that make up the user interface of the addon. Depending on your yarn version the directory files might change here are some other files.
- babel.config.js: This file contains the configuration for Babel, a tool that is used to convert modern JavaScript code into a form that is compatible with older browsers.
- cypress: This directory contains Cypress, a test automation tool that can be used to write and run end-to-end (E2E) tests for your Volto addon.
- Makefile: This file contains a set of commands that can be run from the command line to automate tasks related to building, testing, and deploying your Volto addon.
Developing the addon:
Now that we have a basic understanding of the structure of a Volto addon, let’s start developing our own.
First, let’s create a new React component that will be the main feature of our addon. In the src/views/ directory, create a new file called “HelloWorldView.js” and add the following code:
import React from 'react';
const HelloWorldView = (props) => {
return
HelloWorld!!!!!!;
};
export default HelloWorldView;
This component will simply display the text “Hello, World!” on the screen.
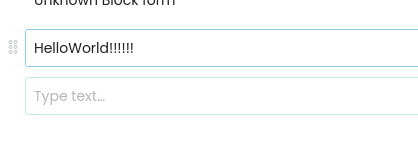
Next, let’s add this component to the user interface of our Plone website. To do this, we need to define a Volto block that will render our component. In the src/views directory, create a new file called “HelloWorldEdit.js” and add the following code:
import React from 'react';
import HellowWorldView from './HelloWorldView';
const HelloWorldEdit = (props) => {
return (
);
};
export default HelloWorldEdit;
Finally, we need to register our block with Volto. In the src/index.js file, add the following code:
import HelloWorldEdit from "./views/HelloWorldEdit";
import HelloWorldView from "./views/HelloWorldView";
import textSVG from '@plone/volto/icons/text.svg';
export default (config) => {
config.blocks.blocksConfig.helloworld = {
id: 'helloworld',
title: 'Hello World',
group: 'common',
icon: textSVG,
view: HelloWorldView,
edit: HelloWorldEdit,
restricted: false,
mostUsed: true,
sidebarTab: 1,
security: {
addPermission: [],
view: [],
},
};
return config;
};
This will register the HelloWorldView block with the “helloWorld” id and “Hello World” title. It will also use the “font” icon and will be available in the “common” group of blocks.
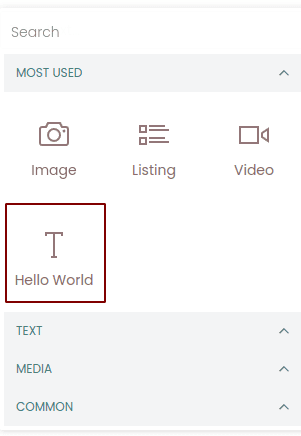
To test our addon, we need to install it in our Plone instance and add the HelloWorld block to a page.
To install the addon:
Add the addon name to package.json (to the root of the project):
"addons": [
"my-addon"
],
After that, make sure to add the addon to jsconfig.json (to the root of the project):
{
"compilerOptions": {
"paths": {
"my-addon":["addons/my-addon/src"]
},
"baseUrl": "src"
}
}
This will build the addon and install it in your Plone instance.
To add the HelloWorld block to a page, log in to the Plone backend and navigate to the page where you want to add the block. Click on the “Add new” button and select the “Hello World” block from the list.
You should now see the “Hello, World!” text displayed on the page.
Volto addons are a powerful way to extend the functionality of Plone and create rich and interactive websites and applications.